Q&A about software engineering
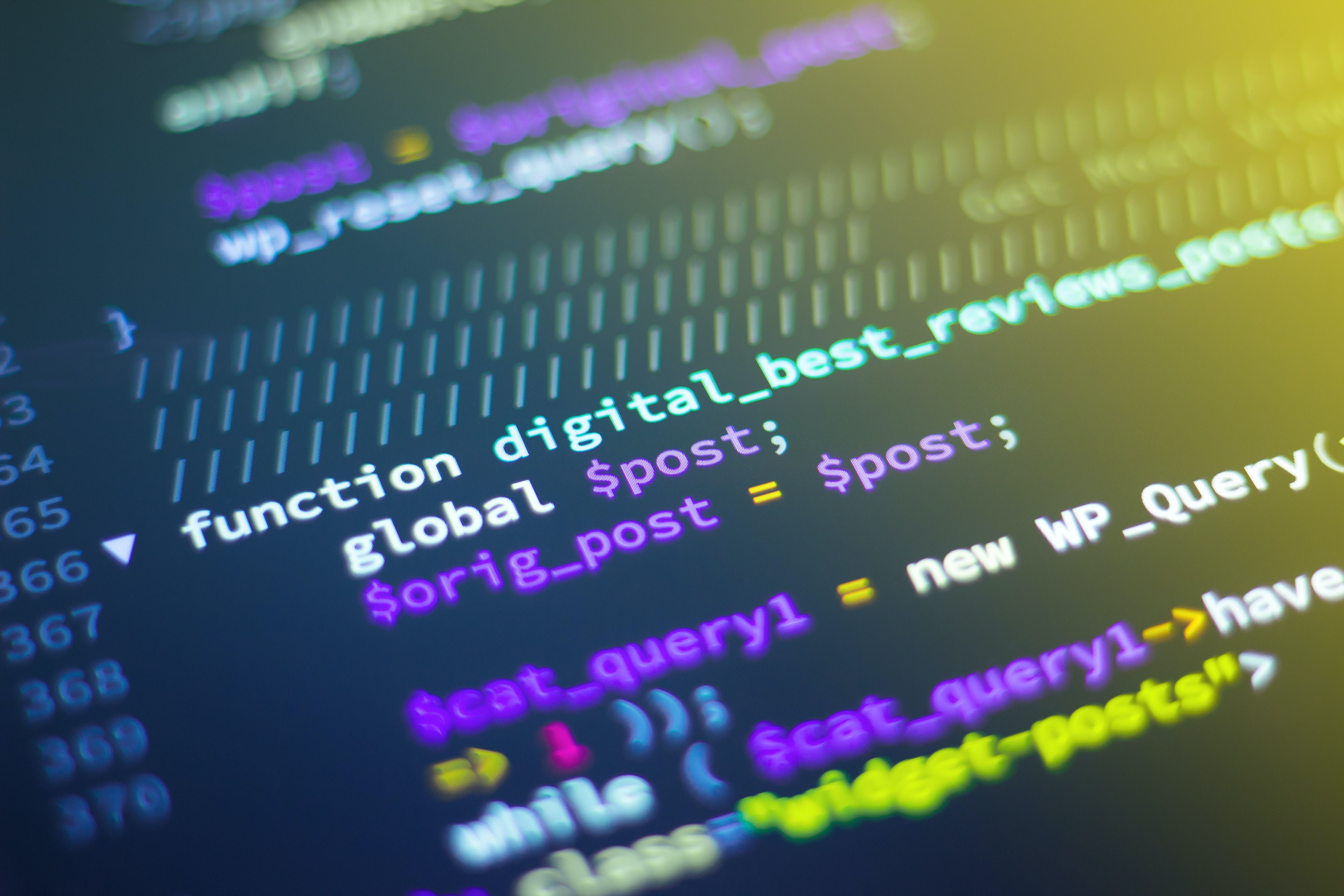
\ o /
How would you identify well-designed object-oriented code?
My favourite quotation regarding this is the following (and I open my coding journal that I keep with the same quote):
"One of the great leaps in OO is to be able to answer the question “How does this work?” with “I don’t care”." (Alan Knight)
Also, not merely regarding OO: "Value = Why / How" (David Hussman)
If these two apply, then I see well designed OO code. In more detail: it is useful if the code is kept SOLID.
A few signs and side effects of that: if the code is maintainable; if I can find my way through the code-base; its components, on various levels of abstraction are interchangeable with alternatives, and only are responsible for what they should be responsible for; if standards in naming, best practices, etc. are kept, then the program is most likely well designed.
Many frameworks endorse a certain layout for proper program structure, readily incorporating OO best practices, that is also helpful.
\ o /
Please explain one of the SOLID OO-Design Principles.
I will stick with the single responsibility principle, which I already touched on earlier, and I will give you a non-textbook explanation.
In my eyes, designing or progressively building a big application is about working with and abstraction of different layers of complexity vertically, and separating responsibilities, almost atomizing in this sense horizontally.
If we keep to the single responsibility principle, then the building blocks of our code remain simple, which enables maintainability. And also means that the code is readable, testable, easily expandable, that each such building block can be used in many ways when necessary.
\ o /
What are the essential properties of object-oriented code?
Maybe the question refers to the four pillars of OO, which are encapsulation, inheritance, polymorphism and abstraction. Extremely briefly:
o Each class "has" all its parts.
o Classes may form a hierarchy in which descendants inherit the qualities of their ancestors (depending on the language and runtime it may be single or multiple).
o For OO, runtime polymorphism is more relevant than compile-time, and it is about using superclasses and subclasses interchangeably in certain scenarios. Compile-time polymorphism also plays a role though because different method signatures within a class with the same method name usually enables a cleaner class structure.
o Abstraction is about defining a structure, and a way of operation for the user of said structure, while enabling multiple execution paths, adaptability and dynamism in the implementation of those functionalities.
\ o /
Please describe one GoF Design Pattern which you recently used and why.
The simplest and widely used pick is the singleton creational pattern. I wrote a web framework where each big "governing" utility class is a singleton. If we need one and only one instance of a class, then it should be a singleton. Gets created upon first use, and maintains state on any further referral, from wherever.
On a personal note, I find myself implementing patterns first, then realizing that it was a pattern - with the exception of those cases where we usually keep the naming of the pattern in the naming of identifiers, e.g. in case of factories.
Once I designed and implemented an interpreted programming language, that must have been the interpreter behavioural pattern.
(I lean to agree with Paul Graham, Peter Norvig, etc. though, that too many patterns used means trouble somewhere deeper, either a shortcoming of the language that we have to overcome or too little abstraction somewhere.)
\ o /
Please describe the meaning of Refactoring in your own words.
Make it better - from the point of view of simplicity, performance, maintainability, clarity, a sense of logical structure, as per the state of the software now. (What might have been logical a while ago, may not be a good fit now.)
The functionality of the program is maintained throughout refactoring.
A possible reason for refactoring might be pulling back the code to the standards mentioned above, if there was a deviation. Which may occur naturally throughout development.
\ o /
What skills do you think our new developers need? What do you think we are currently looking for?
Inferring from the previous questions you are looking for someone whose "programming nature" incorporates the best practices in modern languages, probably on an enterprise scale. Maybe people who can make existing code structure better, or maintain a clean structure. People who can tackle larger complexity regarding interfaces or business logic. The questions did not target specific top-notch tech, rather general principles on which good products can be built.
\ o /
Please describe different (Network) API-Styles e.g. REST.
I have experience with SOAP (protocol) and REST (architecture pattern) so far. Now I am eyeing with combining it with GraphQL as a query language.
Most enterprise apps I worked on used SOAP, and from a developer point of view we had a relatively lucky time with it. (Automatic interface generation, etc.) We benefited from the typed nature of the protocol, states, and the ease of use that WSDL descriptors provided, as a consumer of many external interfaces. Testing was fairly easy with SoapUI as well.
My REST experience is rather seamless so far, generally through frameworks that do it for me. I know it goes through HTTP, uses SSL, I enjoy using it with JSON as opposed to XML (being a fan of human-readability). I am aware of its architectural constraints as well.
I did not have to dig into the depth of neither protocol other than working with professionals who did that for us and designed the systems, I had to only change or extend the services from time to time.
\ o /
Which book would you recommend programmers to read and why?
From the top of my head:
JavaScript: The Good Parts by Douglas Crockford - From his bio: "He is best known for having discovered that there are good parts in JavaScript. That was the first important discovery of the Twenty First Century." That is my main reason for picking this one.
SQL Performance Explained by Markus Winand - because my logic is not fully SQL-compatible, and I would like to help that situation, maybe also a useful volume for others
Fullstack Vue by Hassan Djirdeh - because I am currently reading this one
Bonus: Programming Clojure by Stuart Halloway - because I consider Rich Hickey a genius and if I had more incentive and time to reprogram my brain to pure functional programming, I would do so
\ o /
With regard to our job description, please describe in a few words why you would consider yourself a good fit for this role.
Due to my experience in the field and capability to quickly adapt to various business domains and roles.